Mastering Data Analysis with Pandas: A Comprehensive Guide for Beginners to Advanced Users
- SCHEMOX
- Jan 2, 2024
- 14 min read
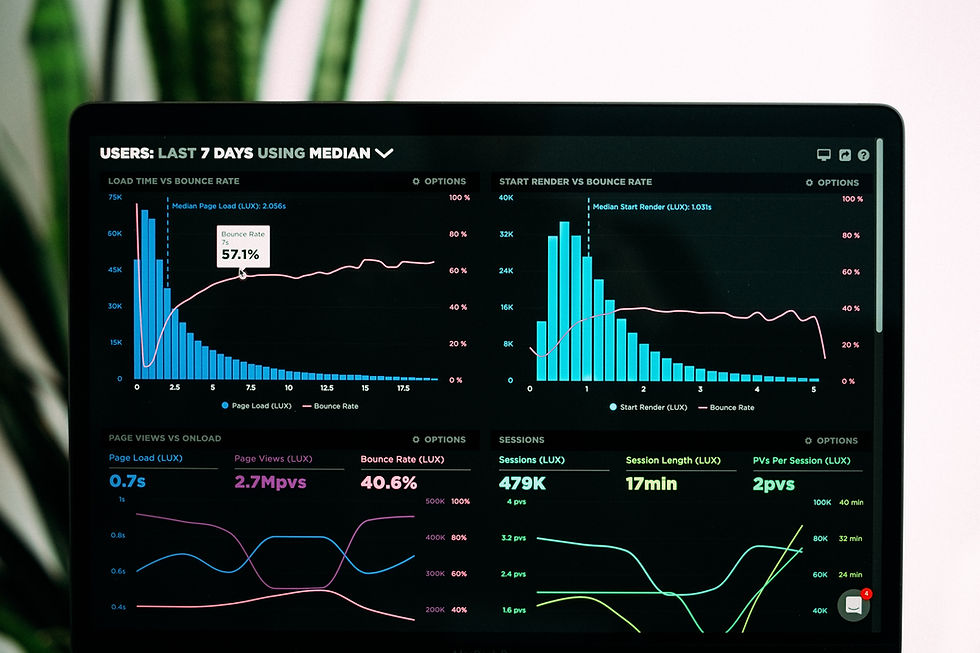
Table of Contents:
4.2 Removing Duplicates
12. Conclusion and Futher Learning
1. Introduction to Pandas
1.1 What is Pandas?
Pandas is an open-source data manipulation and analysis library for Python. Developed by Wes McKinney, it provides easy-to-use data structures like Series and DataFrame, along with a wide range of functions for efficient data manipulation. The name "Pandas" is derived from the term "Panel Data," a type of multidimensional data involving observations over multiple time periods.
Pandas is particularly popular among data analysts, scientists, and machine learning practitioners due to its simplicity and versatility in handling structured data.
1.2 Why use Pandas for Data Analysis?
Pandas simplifies the data analysis process by offering high-level data structures and functions designed for ease of use. Some key reasons to choose Pandas for data analysis include:
Data Structures: Pandas provides two primary data structures - Series and DataFrame. These structures are powerful and flexible, allowing users to handle a wide range of data types and formats.
Data Cleaning: Pandas offers robust tools for cleaning and preprocessing data. It enables users to handle missing data, eliminate duplicates, and apply various transformation functions.
Data Manipulation: Pandas excels in data manipulation tasks such as filtering, sorting, and selecting specific columns or rows. It also supports the application of functions to the entire dataset.
Integration with Other Libraries: Pandas seamlessly integrates with other popular libraries like NumPy, Matplotlib, and Scikit-Learn, providing a comprehensive ecosystem for data analysis and machine learning.
Time Series Analysis: For working with time-series data, Pandas offers specialized tools and functions, making it a valuable tool for financial analysts and researchers.
1.3 Installing Pandas
Before diving into Pandas, you need to install it. You can install Pandas using pip, the Python package installer. Open your terminal or command prompt and run the following command:
pip install pandas
Make sure you have Python installed on your system before running this command.
Once installed, you can start using Pandas in your Python scripts or Jupyter Notebooks.
In the upcoming sections, we'll explore the fundamental aspects of working with Pandas, starting with basic operations and data structures.
2. Getting Started with Pandas
Pandas is a powerful library for data manipulation and analysis, offering two primary data structures: Series and DataFrame. In this section, we'll cover the basics of getting started with Pandas, including importing the library, understanding its fundamental data structures, and performing basic operations.
2.1 Importing Pandas
Before using Pandas, you need to import it into your Python environment. This can be done with a simple import statement:
import pandas as pd
By convention, Pandas is often imported as pd for brevity. This allows you to use pd as a prefix for Pandas functions and classes.
2.2 Pandas Data Structures (Series and DataFrame)
2.2.1 Series
A Series is a one-dimensional array-like object that can hold any data type. It consists of an index and corresponding values. Creating a Series is straightforward:
import pandas as pd
# Creating a Series from a list
data = [1, 2, 3, 4, 5]
series = pd.Series(data)
print(series)
2.2.2 DataFrame
A DataFrame is a two-dimensional table with rows and columns, similar to a spreadsheet or SQL table. It can be thought of as a collection of Series. Creating a DataFrame can be done in various ways, such as from a dictionary or by reading data from an external source:
# Creating a DataFrame from a dictionary
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'San Francisco', 'Los Angeles']}
df = pd.DataFrame(data)
print(df)
2.3 Basic Operations with Pandas
Now that we have our data structures, let's explore some basic operations.
2.3.1 Accessing Elements
You can access elements in a Series or DataFrame using index notation:
# Accessing elements in a Series
print(series[2])
# Accessing elements in a DataFrame
print(df['Name'][1])
2.3.2 Descriptive Statistics
Pandas provides useful functions for obtaining descriptive statistics from your data:
# Descriptive statistics for a Series
print(series.describe())
# Descriptive statistics for a DataFrame
print(df.describe())
These are just the basics, and Pandas offers a wide range of functionality for data manipulation and analysis. In the following sections, we'll delve deeper into loading and inspecting data, data cleaning, and various data manipulation techniques.
3. Data Loading and Inspection
In this section, we'll explore how to load data into Pandas and perform an initial inspection of the dataset. Understanding how to load and inspect data is a crucial first step in any data analysis process.
3.1 Loading Data into Pandas
Pandas supports various file formats for data input, including CSV, Excel, SQL databases, and more. Let's focus on loading data from a CSV file as an example:
import pandas as pd
# Load data from a CSV file
file_path = 'your_data.csv'
df = pd.read_csv(file_path)
Replace 'your_data.csv' with the actual path to your CSV file. Pandas will create a DataFrame (df) containing the data from the CSV file.
3.1.1 Other Data Loading Methods
Excel File:
df_excel = pd.read_excel('your_data.xlsx', sheet_name='Sheet1')
SQL Database:
import sqlite3 conn = sqlite3.connect('your_database.db') query = 'SELECT * FROM your_table' df_sql = pd.read_sql(query, conn)
3.2 Exploring the Dataset
Once the data is loaded, it's essential to perform an initial exploration. Here are some key steps:
3.2.1 Displaying the Head and Tail
# Display the first 5 rows
print(df.head())
# Display the last 5 rows
print(df.tail())
3.2.2 Dataset Information
# Summary information about the DataFrame
print(df.info())
3.2.3 Summary Statistics
# Descriptive statistics for numerical columns
print(df.describe())
3.2.4 Unique Values
# Unique values in a column
print(df['Column_Name'].unique())
3.3 Handling Missing Data
Dealing with missing data is a critical aspect of data analysis. Pandas provides methods to identify and handle missing values:
# Check for missing values in the entire DataFrame
print(df.isnull().sum())
# Drop rows with missing values
df_clean = df.dropna()
# Fill missing values with a specific value
df_fill = df.fillna(value=0)
Understanding these basic data loading and inspection techniques sets the foundation for more advanced data analysis tasks. In the next sections, we'll delve into data cleaning with Pandas and various manipulation techniques.
4. Data Cleaning with Pandas
Data cleaning is a crucial step in the data analysis process. In this section, we'll explore how to handle missing data, remove duplicates, and perform other essential data cleaning tasks using Pandas.
4.1 Handling Missing Data
4.1.1 Identifying Missing Values
Before addressing missing values, it's essential to identify where they exist in the dataset. Pandas provides methods to check for missing values:
# Check for missing values in the entire DataFrame
print(df.isnull().sum())
# Check for missing values in a specific column
print(df['Column_Name'].isnull().sum())
4.1.2 Strategies for Dealing with Missing Values
Dropping Rows with Missing Values:
# Drop rows with missing values
df_clean = df.dropna()
Filling Missing Values:
# Fill missing values with a specific value
df_fill = df.fillna(value=0)
Interpolation:
# Interpolate missing values
df_interpolated = df.interpolate()
4.2 Removing Duplicates
Duplicate rows can skew analysis results. Pandas makes it easy to identify and remove duplicates:
# Identify and remove duplicate rows
df_no_duplicates = df.drop_duplicates()
4.3 Data Imputation Techniques
4.3.1 Mean, Median, or Mode Imputation
Imputing missing values with the mean, median, or mode of the column is a common strategy:
# Impute missing values with the mean
mean_value = df['Column_Name'].mean()
df_imputed_mean = df['Column_Name'].fillna(mean_value)
4.3.2 Forward and Backward Fill
For time-series data, forward or backward fill can be useful:
# Forward fill missing values
df_forward_fill = df.fillna(method='ffill')
# Backward fill missing values
df_backward_fill = df.fillna(method='bfill')
Understanding and applying these data cleaning techniques ensures that the dataset is prepared for meaningful analysis. In the next sections, we'll explore data manipulation with Pandas, including selecting, filtering, and transforming data.
5. Data Manipulation with Pandas
Data manipulation involves selecting, filtering, and transforming data to extract meaningful insights. In this section, we'll explore the powerful capabilities of Pandas for manipulating data in a DataFrame.
5.1 Selecting and Indexing Data
5.1.1 Selecting Columns
You can select one or more columns from a DataFrame using their names:
# Select a single column
column_series = df['Column_Name']
# Select multiple columns
columns_subset = df[['Column1', 'Column2']]
5.1.2 Selecting Rows by Index
You can select specific rows using their index:
# Select rows by index
subset_rows = df.loc[3:6]
5.1.3 Selecting Rows by Condition
Filtering rows based on a condition is a common operation:
# Select rows where a condition is met
conditioned_rows = df[df['Column_Name'] > 50]
5.2 Filtering and Sorting Data
5.2.1 Filtering Data
Filtering data based on specific conditions is crucial for focusing on relevant information:
# Filter data based on a condition
filtered_data = df[df['Column_Name'] > 50]
5.2.2 Sorting Data
Sorting data allows you to arrange it in a meaningful way:
# Sort data by a specific column
sorted_data = df.sort_values(by='Column_Name', ascending=False)
5.3 Applying Functions to Data
5.3.1 Applying Functions to Columns
You can apply functions to entire columns using the apply function:
# Apply a function to a column
df['New_Column'] = df['Old_Column'].apply(lambda x: x * 2)
5.3.2 Applying Functions to Rows
Applying functions to rows can be achieved using the apply function with axis=1:
# Apply a function to each row
df['New_Column'] = df.apply(lambda row: row['Column1'] + row['Column2'], axis=1)
These data manipulation techniques provide the foundation for in-depth analysis and insights. In the upcoming sections, we'll explore advanced topics such as grouping and aggregation, merging and joining DataFrames, and time series analysis with Pandas.
6. Grouping and Aggregation
Grouping and aggregation are powerful operations in Pandas that allow you to analyze data at a higher level of granularity. In this section, we'll explore how to group data based on specific criteria and perform aggregate functions on those groups.
6.1 GroupBy in Pandas
6.1.1 Basic GroupBy Operation
The groupby operation in Pandas involves splitting the data into groups based on some criteria, applying a function to each group independently, and combining the results. Let's consider a simple example:
# Grouping by a single column
grouped_data = df.groupby('Category')
6.1.2 Grouping by Multiple Columns
You can also group by multiple columns to create a hierarchical index:
# Grouping by multiple columns
multi_grouped_data = df.groupby(['Category', 'Subcategory'])
6.2 Aggregating Data
Once you've created groups, you can apply various aggregate functions to summarize the data within each group.
6.2.1 Common Aggregate Functions
Sum:
# Summing values within each group
sum_per_group = grouped_data['Value'].sum()
Mean:
# Calculating the mean within each group
mean_per_group = grouped_data['Value'].mean()
Count:
# Counting the number of entries within each group
count_per_group = grouped_data['Value'].count()
6.2.2 Custom Aggregation Functions
You can also apply custom aggregation functions using the agg method:
# Applying custom aggregation functions
custom_aggregation = grouped_data.agg({'Value': ['sum', 'mean', 'count']})
6.3 Transforming Data
Transformations involve modifying the values within each group without changing the structure of the original DataFrame.
# Applying a transformation within each group
df['Normalized_Value'] = grouped_data['Value'].transform(lambda x: (x - x.mean()) / x.std())
Grouping and aggregation are powerful tools for gaining insights into your data, especially when dealing with large datasets. In the next sections, we'll explore advanced topics such as merging and joining DataFrames to combine information from different sources.
7. Merging and Joining DataFrames
In data analysis, it's common to work with multiple datasets and combine them to derive more comprehensive insights. Pandas provides powerful tools for merging and joining DataFrames, allowing you to bring together data from different sources.
7.1 Combining DataFrames
7.1.1 Concatenation
Concatenation is the process of combining DataFrames along a particular axis, either rows or columns:
# Concatenating DataFrames along rows
concatenated_rows = pd.concat([df1, df2])
# Concatenating DataFrames along columns
concatenated_columns = pd.concat([df1, df2], axis=1)
7.1.2 Append
The append method is a convenient way to add rows to an existing DataFrame:
# Appending rows to a DataFrame
appended_rows = df1.append(df2)
7.2 Merging DataFrames
7.2.1 Inner Merge
An inner merge combines rows that have matching values in both DataFrames:
# Inner merge based on a common column
inner_merged = pd.merge(df1, df2, on='Common_Column')
7.2.2 Left and Right Merge
Left and right merges include all rows from the left or right DataFrame and the matching rows from the other DataFrame:
# Left merge
left_merged = pd.merge(df1, df2, on='Common_Column', how='left')
# Right merge
right_merged = pd.merge(df1, df2, on='Common_Column', how='right')
7.2.3 Outer Merge
An outer merge includes all rows from both DataFrames, filling in missing values with NaN:
# Outer merge
outer_merged = pd.merge(df1, df2, on='Common_Column', how='outer')
7.3 Concatenating and Merging Best Practices
Check for Common Columns: Ensure that the DataFrames to be merged have common columns for merging.
Handle Duplicate Columns: If the DataFrames have columns with the same name, consider renaming them before merging.
Specify the Merge Key: Explicitly specify the key(s) on which to merge using the on parameter.
Handle Missing Values: Be aware of how missing values are handled, especially in different types of merges.
In the next section, we'll explore time series analysis with Pandas, diving into handling dates and times, resampling, and frequency conversion.
8. Time Series Analysis with Pandas
Time series data involves observations recorded over time, and Pandas provides specialized tools for handling and analyzing such data. In this section, we'll explore the features of Pandas that make it a powerful tool for time series analysis.
8.1 Handling Dates and Times
8.1.1 Converting Strings to DateTime
Pandas allows you to convert strings representing dates and times to DateTime objects:
# Convert a column to DateTime
df['Date'] = pd.to_datetime(df['Date'])
8.1.2 Extracting Components
You can extract various components (year, month, day, etc.) from DateTime objects:
# Extracting year, month, and day
df['Year'] = df['Date'].dt.year
df['Month'] = df['Date'].dt.month
df['Day'] = df['Date'].dt.day
8.2 Resampling and Frequency Conversion
8.2.1 Downsampling
Downsampling involves reducing the frequency of the data, typically aggregating over larger intervals:
# Downsampling to monthly frequency
monthly_data = df.resample('M').mean()
8.2.2 Upsampling
Upsampling involves increasing the frequency of the data, often requiring filling or interpolating missing values:
# Upsampling to daily frequency with forward fill
daily_data = df.resample('D').ffill()
8.3 Time Series Visualization
8.3.1 Plotting Time Series Data
Pandas integrates with Matplotlib for convenient time series visualization:
import matplotlib.pyplot as plt
# Plotting a time series
df.plot(x='Date', y='Value', kind='line')
plt.show()
8.3.2 Rolling Windows
Rolling windows allow you to perform operations on a specified window of time:
# Computing a rolling average
df['Rolling_Avg'] = df['Value'].rolling(window=7).mean()
Time series analysis with Pandas opens up opportunities for understanding patterns, trends, and seasonality in your data. In the next section, we'll explore advanced topics in Pandas, including working with categorical data, pivot tables, and memory optimization techniques.
9. Advanced Topics in Pandas
In this section, we'll delve into advanced topics in Pandas that enhance its capabilities for data analysis. These topics include working with categorical data, creating pivot tables, and optimizing memory usage.
9.1 Working with Categorical Data
9.1.1 Introduction to Categorical Data
Categorical data represents data that can take on a limited and usually fixed number of values. Pandas provides a Categorical data type to efficiently work with such data:
# Converting a column to Categorical
df['Category'] = pd.Categorical(df['Category'])
9.1.2 Benefits of Categorical Data
Memory Efficiency: Categorical data often requires less memory compared to the same data represented as strings.
Improved Performance: Operations on Categorical data can be faster than on string data.
Ordered Categories: Categorical data can have an order, which is useful for sorting and comparisons.
9.2 Pivot Tables in Pandas
9.2.1 Creating a Pivot Table
A pivot table is a powerful tool for reshaping and summarizing data:
# Creating a pivot table
pivot_table = df.pivot_table(values='Value', index='Date', columns='Category', aggfunc='mean')
9.2.2 Custom Aggregation Functions
You can use custom aggregation functions in a pivot table:
# Creating a pivot table with a custom aggregation function
pivot_table_custom = df.pivot_table(values='Value', index='Date', columns='Category', aggfunc=lambda x: x.max() - x.min())
9.3 Memory Optimization Techniques
9.3.1 Convert Numeric Columns to Appropriate Types
Ensure numeric columns are of the appropriate type to optimize memory usage:
# Convert numeric columns to appropriate types
df['Numeric_Column'] = pd.to_numeric(df['Numeric_Column'], downcast='float')
9.3.2 Use Sparse Data Structures
For datasets with a significant number of missing values, consider using sparse data structures:
# Convert DataFrame to Sparse DataFrame
sparse_df = df.to_sparse()
9.3.3 Downcast Integer Columns
Downcast integer columns to reduce memory usage:
# Downcast integer columns
df['Integer_Column'] = pd.to_numeric(df['Integer_Column'], downcast='integer')
Understanding these advanced topics in Pandas allows for more efficient and sophisticated data analysis. In the concluding section, we'll recap key concepts and outline the next steps in your data analysis journey.
10. Case Study: Real-world Data Analysis
In this section, we'll apply the knowledge gained throughout this guide to a real-world case study. We'll identify a real-world dataset, load and preprocess the data, perform exploratory data analysis (EDA), and draw insights and conclusions. This case study will showcase the practical application of Pandas for in-depth data analysis.
10.1 Identifying a Real-world Dataset
Choose a dataset relevant to your interests or the focus of your analysis. Consider datasets available from reputable sources such as Kaggle, government databases, or research institutions. For example, you might choose a dataset related to finance, healthcare, social trends, or any field of interest.
10.2 Loading and Preprocessing Data
10.2.1 Loading the Dataset
Load the chosen dataset into a Pandas DataFrame:
import pandas as pd
# Load the dataset
df = pd.read_csv('your_dataset.csv')
10.2.2 Initial Exploration
Perform an initial exploration of the dataset to understand its structure and contents:
# Display the first few rows of the dataset
print(df.head())
# Summary information about the DataFrame
print(df.info())
10.3 Exploratory Data Analysis (EDA)
10.3.1 Descriptive Statistics
Calculate and analyze descriptive statistics to gain insights into the dataset:
# Descriptive statistics for numerical columns
print(df.describe())
10.3.2 Data Visualization
Visualize key aspects of the data using plots and charts:
import matplotlib.pyplot as plt
import seaborn as sns
# Plotting distribution of a numerical variable
sns.histplot(df['Numeric_Column'], bins=20)
plt.title('Distribution of Numeric_Column')
plt.show()
10.4 Drawing Insights and Conclusions
Based on the exploratory data analysis, draw insights and conclusions about the dataset. Consider patterns, trends, correlations, and potential areas for further investigation.
11. Best Practices and Tips
In this section, we'll cover best practices and tips for working effectively with Pandas. Following these guidelines can enhance the efficiency, readability, and reliability of your code.
11.1 Writing Efficient Pandas Code
11.1.1 Use Vectorized Operations
Take advantage of Pandas' vectorized operations instead of using loops for element-wise operations:
# Avoid using loops for element-wise operations
df['New_Column'] = df['Old_Column'] * 2
11.1.2 Utilize the apply Function Judiciously
While the apply function is powerful, use it judiciously, as it can be computationally expensive. Vectorized operations are often more efficient.
# Use apply for custom operations, but be mindful of performance
df['New_Column'] = df['Column'].apply(lambda x: custom_function(x))
11.2 Handling Large Datasets
11.2.1 Use chunksize Parameter for Large File Reading
When dealing with large datasets that don't fit into memory, use the chunksize parameter while reading files to process data in smaller chunks:
# Reading a large CSV file in chunks
chunk_size = 10000
for chunk in pd.read_csv('large_data.csv', chunksize=chunk_size):
process(chunk)
11.2.2 Use 'nrows' Parameter for Initial Exploration
For initial exploration of large datasets, use the nrows parameter to read only a subset of rows:
# Initial exploration of a large dataset with limited rows
subset_df = pd.read_csv('large_data.csv', nrows=1000)
11.3 Common Pitfalls and How to Avoid Them
11.3.1 Beware of SettingWithCopy Warnings
Be cautious about settingWithCopy warnings. When assigning new values based on a condition, use the copy method to avoid potential issues:
# Avoid settingWithCopy warning
df_subset = df[df['Column'] > 50].copy()
11.3.2 Carefully Handle Missing Data
Handle missing data thoughtfully, considering the impact on analysis. Use appropriate methods such as imputation or dropping rows based on the context.
# Handle missing data appropriately
df_clean = df.dropna()
11.4 Documentation and Comments
11.4.1 Document Your Code
Provide clear documentation for your code, especially if you're working on a collaborative project or sharing your code with others:
"""
This function performs a custom operation on the DataFrame.
Parameters:
- df (DataFrame): Input DataFrame.
- column_name (str): Name of the column to be processed.
Returns:
- DataFrame: Processed DataFrame.
"""
def custom_operation(df, column_name):
# Code implementation here
return processed_df
11.4.2 Use Informative Variable and Column Names
Choose meaningful and descriptive variable and column names to enhance code readability:
# Use descriptive variable and column names
total_sales = df['Revenue'].sum()
Following these best practices and tips can contribute to writing cleaner, more efficient, and maintainable Pandas code. It also helps in avoiding common pitfalls and ensuring that your data analysis workflows are robust and reliable.
12. Conclusion and Further Learning
Congratulations on completing this comprehensive guide to Pandas for data analysis! You've gained essential skills in loading, cleaning, manipulating, and analyzing data using Pandas, a powerful library in the Python ecosystem.
12.1 Recap of Key Learnings
Let's recap some key learnings from this guide:
Data Loading: You've learned how to load data from various sources, including CSV files, Excel sheets, SQL databases, and more.
Data Cleaning: The guide covered techniques for handling missing data, removing duplicates, and transforming data to ensure its quality and reliability.
Data Manipulation: You explored how to select, filter, and transform data, enabling you to extract meaningful insights.
Grouping and Aggregation: You learned how to group data based on specific criteria and perform aggregate functions to summarize information.
Merging and Joining DataFrames: The guide covered concatenation, as well as various types of merges, providing flexibility in combining data from different sources.
Time Series Analysis: You gained insights into handling dates and times, resampling, and frequency conversion, which are crucial for analyzing time series data.
Advanced Topics: This section covered advanced topics such as working with categorical data, creating pivot tables, and optimizing memory usage for efficient data analysis.
12.2 Applying Skills in Real-world Projects
Now that you have a solid understanding of Pandas, consider applying your skills in real-world projects. Choose a domain or industry of interest and explore datasets related to that field. Practice your skills by conducting exploratory data analysis, drawing insights, and presenting findings.
12.3 Further Learning Resources
If you're eager to deepen your knowledge, consider exploring the following resources:
Official Pandas Documentation: The official documentation is an excellent resource for detailed information and examples: Pandas Documentation
Online Courses: Platforms like Coursera, Udemy, and DataCamp offer courses specifically focused on Pandas and data analysis.
Books: Books like "Python for Data Analysis" by Wes McKinney, the creator of Pandas, provide in-depth insights into practical data analysis using Pandas.
Community Forums: Engage with the data science community on forums like Stack Overflow and the Pandas Google Group. You can learn from others' experiences and get help with specific challenges.
12.4 Keep Exploring and Learning
Data analysis is a dynamic field, and technologies evolve. Keep exploring new tools, libraries, and techniques to stay current. Whether you're interested in machine learning, data visualization, or other data-related domains, the skills you've developed with Pandas serve as a solid foundation for your data science journey.
Thank you for joining this learning journey. I wish you continued success in your data analysis endeavors!
Opmerkingen