Mastering HTML: A Comprehensive Guide to Web Development Essentials and Beyond
- SCHEMOX
- Sep 16, 2023
- 30 min read
Updated: Sep 17, 2023

Table of contents
Introduction to HTML
HTML Forms and Input Elements
Working with Multimedia
Styling with CSS
HTML5 and Modern Web Features
Web Accessibility
Advanced HTML Topics
JavaScript and HTML Interaction
Building a Complete Web Page
Conclusion and Further Learning
Introduction to HTML
HTML, or Hypertext Markup Language, is the backbone of the World Wide Web. It serves as the standard markup language used to create web pages. In this article, we will delve into the fundamental aspects of HTML, understanding what it is, exploring its building blocks, and dissecting the anatomy of an HTML document.
1.1 What is HTML?
HTML stands for Hypertext Markup Language. It is a standardized system of tags and attributes used to create documents on the World Wide Web. HTML documents are the building blocks of web pages, and they are interpreted by web browsers to display content to users.
HTML is not a programming language; it is a markup language. This means that HTML is used to structure the content on a web page, defining elements such as headings, paragraphs, lists, links, and more. It provides a way to organize information so that it can be presented in a structured and meaningful manner.
HTML documents are plain text files with a .html file extension. They can be created using a simple text editor or specialized HTML editors.
1.2 The Building Blocks of HTML
HTML documents are constructed using a set of fundamental building blocks known as elements. These elements are the essential components that structure and define the content of a web page. Each element is enclosed within HTML tags, which are essentially markers that tell the web browser how to render and display the content. Here's a closer look at these HTML building blocks:
1.2.1 HTML Tags
HTML tags are the core of HTML markup. They are used to enclose and define the beginning and end of elements. Tags are enclosed within angle brackets (< and >), and most elements consist of an opening tag and a closing tag. For instance, to create a paragraph of text, you would use the <p> tag to open the paragraph and </p> to close it:
<p>This is a paragraph of text.</p>
The opening tag (<p>) signifies the beginning of the paragraph, while the closing tag (</p>) indicates its end. This structure is fundamental in ensuring that web browsers can understand and render the content correctly.
1.2.2 Common HTML Elements
HTML offers a wide range of elements to structure and format content. Here are some commonly used ones:
<html>: The root element that encapsulates the entire web page.
<head>: Contains metadata about the document, such as the character encoding and the page's title.
<title>: Sets the title of the web page, displayed in the browser's title bar or tab.
<body>: Houses the primary content of the web page, including text, images, and multimedia.
Headings (<h1>, <h2>, <h3>, etc.): Define hierarchical headings, with <h1> being the highest level and <h6> the lowest.
Paragraphs (<p>): Create blocks of text.
Lists (<ul>, <ol>, <li>): Define unordered (bulleted) or ordered (numbered) lists and list items.
Hyperlinks (<a>): Create clickable links to other web pages or resources.
Images (<img>): Embed images into the web page.
Forms (<form>): Define interactive input forms for user input and data submission.
Tables (<table>, <tr>, <td>): Structure tabular data.
Divs (<div>): A container element used to group and structure content within a web page. It is often styled using CSS to create layout structures.
Spans (<span>): An inline element used to apply styles or scripting to a specific portion of text or content within another element.
1.2.3 HTML Attributes
HTML elements can also have attributes that provide additional information or modify their behaviour. Attributes are specified within the opening tag of an element. For example, the <a> element (used for links) has an href attribute to specify the destination URL:
<a href="https://www.example.com">Visit Example.com</a>
In this example, the 'href' attribute determines where the link points.
1.3 The Anatomy of an HTML Document
Understanding the structure of an HTML document is crucial for creating well-organized and semantically meaningful web pages. An HTML document follows a specific structure to ensure proper interpretation by web browsers. Let's dissect the various components of this structure in greater detail:
1.3.1 Document Type Declaration
At the very beginning of an HTML document, you'll typically find the Document Type Declaration, commonly referred to as <!DOCTYPE>. This declaration serves a critical role in specifying the document type and version of HTML being used. For modern web development, HTML5 is the standard, and the declaration looks like this:
<!DOCTYPE html>
This declaration tells the browser that the document is written in HTML5, enabling modern features and rendering standards.
1.3.2 The HTML Element
The <html> element serves as the root of every HTML document. It encloses the entire content of the page, defining the document's scope. Everything within an HTML document is contained within this opening <html> tag and its corresponding closing <html> tag.
<html>
<!-- All content goes here -->
</html>
1.3.3 The Head Element
Inside the <html> element, the <head> section is reserved for metadata and resource declarations that don't appear directly on the webpage but provide crucial information to browsers and search engines. Common elements found within the <head> include:
<meta charset="UTF-8">: Specifies the character encoding for the document, ensuring proper text rendering.
<title>: Sets the title of the web page, which appears in the browser's title bar or tab.
Links to external resources like stylesheets and scripts.
<head>
<meta charset="UTF-8">
<title>Document Title</title>
<!-- Other metadata and resource links -->
</head>
1.3.4 The Body Element
The <body> element contains the main content of the web page, including text, images, links, and other media. It defines what users see and interact with when they visit your site. All visible elements, from headings and paragraphs to images and lists, reside within the <body> section.
<body>
<h1>Main Heading</h1>
<p>This is a paragraph of text.</p>
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
<!-- Additional content -->
</body>
In essence, the <body> element is where the magic happens. It's where you structure and present your content to create a meaningful and engaging user experience.
Understanding the anatomy of an HTML document is fundamental for web developers and content creators. It provides the foundation for crafting well-structured and visually appealing web pages that are compatible with various browsers and devices.
2. HTML Forms and Input Elements
In the realm of web development, HTML forms are pivotal for interaction and data collection from users. Understanding how to construct and manipulate these forms is a fundamental skill. In this article, we will explore HTML forms and their input elements comprehensively.
2.1 Building Forms
At the core of user interactivity lies the HTML <form> element. It serves as a container for various input elements, allowing users to enter and submit data to web servers. Let's delve into the basics of building HTML forms.
To create a form, you start with the <form> tag. It encloses all the form elements you want to include. The 'action' attribute specifies the URL where the form data will be sent upon submission. The 'method' attribute defines how data is sent, typically as a GET or POST request.
Here's a simple form example:
<form action="/submit" method="post">
<!-- Form elements go here -->
</form>
Within the form, you can add various input elements, which allow users to provide data. These input elements include text inputs, textareas, radio buttons, checkboxes, and select dropdowns.
2.2 Text Inputs and Textareas
Text inputs (<input type="text">) are commonly used for single-line text input. They are suitable for fields like names, email addresses, or search queries.
Textareas (<textarea>) are used for multi-line text input, making them ideal for comments, messages, or longer textual content. You can specify the number of rows and columns to control the textarea size.
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<label for="message">Message:</label>
<textarea id="message" name="message" rows="4" cols="50"></textarea>
2.3 Radio Buttons and Checkboxes
Radio buttons (<input type="radio">) and checkboxes (<input type="checkbox">) allow users to make selections from predefined options. Radio buttons are used when users can choose only one option from a list, while checkboxes allow multiple selections.
To group radio buttons, use the same 'name' attribute value, which ensures that only one option can be selected within that group. Checkboxes can have unique 'name' attributes for independent selections.
<label>Gender:</label><inputtype="radio" id="male" name="gender" value="male"><labelfor="male">Male</label>
<inputtype="radio" id="female" name="gender" value="female">
<labelfor="female">Female</label>
<labelfor="subscribe">Subscribe to Newsletter:</label><inputtype="checkbox" id="subscribe" name="subscribe" value="yes">
2.4 Select Dropdowns and Options
Select dropdowns (<select>) provide users with a list of options, and they can choose one. Each option is defined using the <option> element within the <select> element.
<label for="country">Country:</label>
<select id="country" name="country">
<option value="us">United States</option>
<option value="ca">Canada</option>
<option value="uk">United Kingdom</option>
</select>
2.5 Form Submission and Handling
Once users have filled in the form, they can submit their data. The form's 'action' attribute specifies the URL where the data will be sent. The method of submission (GET or POST) is defined by the method attribute.
Upon submission, the data can be processed by a server-side script, such as PHP or Python, which can handle the input, validate it, and perform necessary actions based on the user's submission.
Whether you are building a simple contact form or a complex data-entry system, mastering HTML forms is a crucial step in creating dynamic and user-friendly web applications.
3. Working with Multimedia
In today's digital landscape, multimedia plays a pivotal role in engaging and enriching web content. From embedding audio and video to creating dynamic graphics, this article explores the world of multimedia in web development.
3.1 Embedding Audio and Video
Audio on the Web
Audio content can be a powerful addition to your website, whether it's for background music, podcasts, or narrations. HTML5 introduced native support for audio playback through the <audio> element. This element allows you to embed audio files directly into web pages, making them accessible to users with ease.
Here's an example of how to embed audio:
<audio controls><source src="audio.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
In this code, the <audio> element includes a <source> element that specifies the audio file's source (in this case, an MP3 file). The 'controls' attribute adds playback controls to the audio player, allowing users to play, pause, and adjust the volume.
The message "Your browser does not support the audio/video element." serves as a fallback or alternative content. It is displayed when a web browser lacks support for the <audio> /<video> element or is unable to play the specified audio format.
Video on the Web
Similarly, HTML5 provides native support for embedding videos using the <video> element. This element enables seamless integration of video content into web pages, enhancing user engagement.
Here's an example of how to embed a video:
<video controls><source src="video.mp4" type="video/mp4">
Your browser does not support the video element.
</video>
Like with audio, the <video> element uses the <source> element to specify the video file source (MP4 in this case) and includes playback controls using the 'controls' attribute.
3.2 The <canvas> Element for Graphics
Creating Dynamic Graphics
The <canvas> element is a dynamic powerhouse for creating graphics directly within web pages using JavaScript. It provides a blank slate where you can draw shapes, images, and even animations.
Here's a basic example of a canvas element:
<canvas id="myCanvas" width="400" height="200"></canvas>
To draw on the canvas, you'll need JavaScript. Here's a simple script that draws a blue rectangle:
<canvas id="myCanvas" width="400" height="200" style="background:black;"></canvas>
<script>const canvas = document.getElementById('myCanvas');
const context = canvas.getContext('2d');
context.fillStyle = 'blue';
context.fillRect(150, 50, 100, 100);
</script>
The script first gets the canvas element by its 'id', retrieves the 2D drawing context, and then draws a blue rectangle.
Animations and Interactivity
The <canvas> element is not limited to static graphics; it can also be used to create interactive animations and games. By updating the canvas in real-time using JavaScript, you can craft dynamic user experiences that respond to user input.
3.3 Optimizing Multimedia for the Web
File Formats and Compression
Optimizing multimedia for the web is crucial for providing a smooth and efficient user experience. Here are some key considerations:
File Formats: Choose appropriate file formats for audio and video. Common choices include MP3 and MP4, which offer good compression and compatibility.
Compression: Compress multimedia files to reduce their size without sacrificing quality. Various tools and codecs are available to achieve this.
Responsive Design: Ensure that multimedia elements are responsive and adapt to different screen sizes and devices. Use CSS and media queries to control their presentation.
Accessibility
Making multimedia content accessible to all users is essential. Provide alternative text descriptions for images, captions for videos, and transcripts for audio content. This ensures that your multimedia is usable by individuals with disabilities and enhances your website's inclusivity.

4. Styling with CSS
In the realm of web development, CSS (Cascading Style Sheets) stands as the design wizard that transforms plain HTML content into visually appealing and organized web pages. This article serves as your guide to understanding the power and versatility of CSS.
4.1 Introduction to CSS
What is CSS?
CSS, or Cascading Style Sheets, is a stylesheet language used to describe the presentation of a document written in HTML or XML. It enables web developers to control the layout, design, and appearance of web pages. CSS separates content from presentation, allowing for a clear distinction between the structure of a web page (HTML) and its visual styling (CSS).
The Importance of CSS
CSS is integral to web development for several reasons:
Enhanced User Experience: CSS makes web pages visually appealing and user-friendly. It allows for the creation of attractive layouts, fonts, colours, and responsive designs.
Consistency: CSS promotes consistency across a website. By defining styles in a central stylesheet, you can ensure that all pages maintain a unified look and feel.
Efficiency: CSS allows you to make global changes to the appearance of a website by editing a single stylesheet. This saves time and effort when updating the design.
Accessibility: CSS can be used to improve web accessibility by providing alternative styles for users with disabilities.
4.2 Inline, Internal, and External CSS
Inline CSS
Inline CSS involves styling elements directly within the HTML document using the style attribute. While it provides immediate styling, it's generally discouraged for larger projects due to its limited maintainability and scalability.
<p style="color: blue; font-size: 18px;">This is a styled paragraph.</p>
Internal CSS
Internal CSS is placed within the HTML document's <head> section using the <style> element. It applies styles to the entire document or specific elements.
<head>
<style>
p {
color: green;
font-size: 16px;
}
</style>
</head>
<body>
<p>This is another styled paragraph.</p>
</body>
External CSS
External CSS is the most widely used method. It involves creating a separate .css file and linking it to the HTML document using the <link> element. This method promotes maintainability and allows for reusing styles across multiple web pages.
<head>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
4.3 Selectors and Styles
CSS Selectors
Selectors in CSS determine which elements on a web page will be styled. There are various types of selectors:
Element Selectors: Target specific HTML elements. For example, p selects all paragraphs.
Class Selectors: Begin with a period (.) and target elements with a specific class attribute. For example, '.highlight' targets all elements with the class "highlight".
ID Selectors: Begin with a hash (#) and target a specific element with a unique ID attribute. For example, '#header' targets an element with the ID "header".
CSS Styles
Styles in CSS define how selected elements should be presented. They include properties and values. For instance:
p {
color: blue;
font-size: 18px;
}
In this example, the colour property sets the text colour to blue, and the font-size property defines the font size.
4.4 CSS Box Model and Layout
Understanding the Box Model
The CSS Box Model is a fundamental concept that defines how elements are rendered on a web page. It consists of four parts: content, padding, border, and margin. Each part contributes to the element's overall dimensions and spacing.
Content: The actual content of the element, such as text or images.
Padding: The space between the content and the element's border.
Border: The boundary that separates the element's content from its padding and margin.
Margin: The space outside the element, creates separation from neighbouring elements.
Layout with CSS
CSS offers multiple techniques for controlling the layout of web pages. Two widely used methods are:
Floats: Elements can be floated left or right within a parent container. This is commonly used for creating multi-column layouts.
Positioning: Elements can be precisely positioned using properties like position, top, right, bottom, and left. This method allows for fine-grained control over element placement.
4.5 CSS Flexbox and Grid Layouts
CSS Flexbox
Flexbox is a powerful layout model that simplifies the arrangement of elements in a flexible and efficient way. It's particularly useful for creating complex layouts with variable content sizes. Flexbox containers can distribute space and align items with ease.
.container {
display: flex;
justify-content: space-between;
align-items: center;
}
CSS Grid Layout
CSS Grid Layout is a two-dimensional layout system that provides precise control over both rows and columns. It excels at creating grid-based designs, such as those seen in magazine layouts or complex web applications.
.container {
display: grid;
grid-template-columns: 1fr 2fr 1fr;
grid-gap: 20px;
}
5. HTML5 and Modern Web Features
In the ever-evolving landscape of web development, HTML5 has emerged as a powerful and versatile markup language. It introduced a host of new features and capabilities that have revolutionized web content creation. This article explores some of the key modern web features of HTML5 and how they enhance the web experience.
5.1 Semantic HTML5 Elements
The Power of Semantics
HTML5 brought a fresh perspective on structuring web content with a set of semantic elements. Unlike their non-semantic counterparts, these elements carry meaning, making the content more accessible to both browsers and humans.
<header>, <nav>, and <footer> Elements
These elements define the header, navigation menu, and footer of a web page. They provide a clear structure for these important sections, aiding in navigation and search engine optimization.
<header>
<h1>Website Title</h1>
</header>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
<footer>
© 2023 Website Name
</footer>
<article> and <section> Elements
These elements are used to define self-contained content sections within a page. The <article> element represents independent content that can stand alone, like a blog post or news article. <section>, on the other hand, groups related content together.
<article>
<h2>Exploring HTML5 Semantics</h2>
<p>HTML5 semantic elements make content more structured...</p>
</article>
<section>
<h3>Benefits of Semantics</h3>
<p>Improved accessibility, SEO, and code readability...</p>
</section>
5.2 Geolocation and Maps
Location-Aware Web Apps
HTML5 introduced the Geolocation API, enabling web applications to access a user's geographical location. This feature has immense potential for location-based services and applications.
if ("geolocation" in navigator) {
navigator.geolocation.getCurrentPosition(function(position) {
const latitude = position.coords.latitude;
const longitude = position.coords.longitude;
// Use the latitude and longitude data...
});
} else {
// Geolocation not supported
}
Embedding Maps
The integration of maps into web pages has become simpler with HTML5. Services like Google Maps provide easy-to-embed code that allows developers to include interactive maps on their websites.
<iframe src="https://www.google.com/maps/embed?pb=!1m14!1m12!1m3!1d78753783.31634316!2d49.49527854040529!3d14.338563275376844!2m3!1f0!2f0!3f0!3m2!1i1024!2i768!4f13.1!5e1!3m2!1sen!2sde!4v1694859153715!5m2!1sen!2sde" width="600" height="450" style="border:0;" allowfullscreen="" loading="lazy"></iframe>
5.3 Local Storage and Web Storage
Client-Side Data Storage
HTML5 introduced client-side storage options, making it possible to store data locally within a user's browser. This is particularly useful for caching, saving preferences, and offline web applications.
Local Storage
Local Storage provides a simple key-value storage mechanism. Data stored in Local Storage remains available even after the user closes the browser.
localStorage.setItem("username", "john_doe");
const storedUsername = localStorage.getItem("username");
Web Storage (Session Storage)
Session Storage is similar to Local Storage but has a shorter lifespan. Data stored in Session Storage is available only for the duration of a page session and is cleared when the session ends.
sessionStorage.setItem("cartTotal", "100");
const total = sessionStorage.getItem("cartTotal");
5.4 Web Workers and Multithreading
Parallel Processing in the Browser
Web Workers bring multithreading capabilities to web applications, allowing them to perform CPU-intensive tasks without blocking the main thread. This keeps the web page responsive and improves the overall user experience.
Creating a Web Worker
// main.js
const worker = new Worker("worker.js");
worker.postMessage("Start processing!");
// worker.js
self.onmessage = function(event) {
const data = event.data;
// Perform time-consuming tasks...
self.postMessage("Processing complete!");
}
Web Workers can handle tasks like image processing, data manipulation, and more, all without affecting the user interface's performance.
6. Web Accessibility
Web accessibility is an essential aspect of modern web development, ensuring that websites are usable by people with disabilities. It's not only a legal and ethical requirement but also a way to enhance the user experience for all. In this comprehensive guide, we'll explore the principles and techniques behind web accessibility.
6.1 Understanding Accessibility
What is Web Accessibility?
Web accessibility, often abbreviated as A11Y (A-eleven-Y), refers to the practice of designing and developing websites and web applications in a way that makes them accessible to everyone, including people with disabilities. Disabilities in this context encompass a wide range of conditions, such as:
Visual impairments
Hearing impairments
Motor impairments
Cognitive impairments
Web accessibility aims to ensure that people with these disabilities can perceive, understand, navigate, and interact with websites effectively.
Why Web Accessibility Matters
Inclusivity: Accessible websites ensure that everyone, regardless of their abilities, can access and use web content. It promotes inclusivity and equal opportunities on the Internet.
Legal and Regulatory Compliance: Many countries have laws and regulations that require websites to be accessible. Non-compliance can result in legal consequences.
Improved SEO: Accessible websites often have better search engine rankings, as search engines value well-structured and semantic content, which is a common aspect of accessible design.
Enhanced User Experience: Accessibility features often enhance the user experience for all users. For example, captions on videos benefit not only the deaf but also those in noisy environments.
6.2 Semantic HTML for Accessibility
The Role of Semantic HTML
Semantic HTML refers to the use of HTML elements that carry meaning about the structure and content of a web page. It is a cornerstone of web accessibility because it provides a clear and meaningful structure that aids in the interpretation of web content by assistive technologies, such as screen readers.
Headings (<h1> to <h6>)
Headings define the hierarchical structure of a web page. Properly nesting headings and using them appropriately helps screen reader users navigate and understand the content.
<h1>Main Heading</h1>
<h2>Subheading</h2>
<p>Content goes here...</p>
Lists (<ul>, <ol>, <li>)
Lists are essential for organizing content. They are used not only for readability but also to provide context to screen reader users.
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
Links (<a>)
Links should have descriptive text that indicates their purpose. Avoid using vague link text like "click here" or "read more."
<a href="/contact">Contact Us</a>
6.3 ARIA Roles and Attributes
ARIA: Accessible Rich Internet Applications
ARIA (Accessible Rich Internet Applications) is a set of attributes that can be added to HTML elements to provide additional information to assistive technologies. It helps make complex web applications more accessible.
Roles
ARIA roles define the type of element and its function. For example, you can use role="button" to indicate that an element functions as a button.
<div role="button" tabindex="0" onclick="doSomething()">Click me</div>
States and Properties
ARIA attributes like aria-checked and aria-hidden convey information about the current state or properties of an element. For instance, aria-hidden="true" hides an element from screen readers.
<button aria-pressed="false" onclick="toggleState(this)">Toggle</button>
6.4 Testing and Auditing Web Accessibility
Ensuring Accessibility Compliance
Creating an accessible website is an ongoing process that involves testing and auditing. Here are some essential steps:
Automated Testing
Use accessibility testing tools and validators to catch common issues. Tools like axe, WAVE, and Lighthouse can help identify accessibility violations.
Manual Testing
Manual testing involves using assistive technologies like screen readers and keyboard navigation to ensure that your website is navigable and understandable for all users.
User Testing
Engage with users who have disabilities to gather feedback and make improvements based on their real-world experiences.
Auditing
Regularly audit your website for accessibility compliance, especially when adding new features or content.
7. Advanced HTML Topics
HTML, the backbone of the web, offers more than just basic markup. In this exploration of advanced HTML topics, we delve into techniques and features that take your web development skills to the next level.
7.1 Custom Data Attributes
Extending HTML with Data Attributes
Custom data attributes, often referred to as "data-*" attributes, allow developers to store extra information directly in HTML elements. These attributes are particularly useful when you need to attach data to elements for scripting, styling, or other purposes without affecting the visual rendering.
Syntax
The syntax for creating a custom data attribute is straightforward:
<div data-custom-info="42">This element has custom data.</div>
In this example, data-custom-info is a custom data attribute that stores the value "42."
JavaScript Interaction
JavaScript can access and manipulate custom data attributes easily:
const element = document.querySelector('div');
const customInfo = element.dataset.customInfo; // Retrieves the value
element.dataset.customInfo = 'New Value'; // Sets a new value
Custom data attributes provide a convenient way to associate data with elements, simplifying scripting and enhancing the maintainability of your code.
7.2 Meta Tags for SEO
Harnessing Meta Tags for Search Engines
Search engine optimization (SEO) is crucial for ensuring that your web content is discoverable by search engines like Google, Bing, and Yahoo. Properly structured meta tags can significantly impact your website's visibility in search results.
Title Tag
The <title> tag defines the title of a web page. It is displayed as the headline in search engine results.
<head>
<title>Best Practices for SEO</title>
</head>
Meta Description
The <meta name="description"> tag provides a brief summary of the page's content. It appears in search results beneath the title.
<meta name="description" content="Learn best practices for optimizing your website's SEO and improving search engine rankings.">
Meta Keywords (Less Important)
In the past, meta keywords were used for SEO, but they are no longer a significant factor in search engine ranking algorithms. However, including them doesn't hurt.
<meta name="keywords" content="SEO, search engine optimization, web development, keywords">
Canonical Tag
The <link rel="canonical"> tag specifies the preferred version of a web page when multiple versions with similar content exist. It helps prevent duplicate content issues.
<link rel="canonical" href="https://www.example.com/your-page">
Robots Meta Tag
The <meta name="robots"> tag instructs search engine crawlers on how to index and follow links on a page. Common values include "index", "follow", "noindex", and "nofollow."
<meta name="robots" content="index, follow">
Properly configured meta tags can significantly improve your website's search engine ranking and visibility.
7.3 Responsive Web Design
Designing for All Devices
In today's digital landscape, ensuring that your website is accessible and usable on various devices and screen sizes is paramount. Responsive web design is the practice of creating web layouts that adapt gracefully to different screen sizes, from large desktop monitors to small mobile devices.
Media Queries
Media queries are a fundamental part of responsive design. They allow you to apply different CSS styles based on the characteristics of the user's device, such as screen width.
@media screen and (max-width: 600px) {
/* Styles for screens with a width of 600px or less */
}
Fluid Layouts
Designing with fluid layouts involves using relative units (e.g., percentages) instead of fixed units (e.g., pixels) for sizing elements. This allows content to adapt smoothly to various screen sizes.
.container {
width: 80%; /* Relative width */
}
Flexbox and Grid
CSS Flexbox and Grid Layout are powerful tools for creating responsive designs. They offer precise control over layout and alignment, making it easier to create complex responsive interfaces.
/* Flexbox example */
.container {
display: flex;
justify-content: space-between;
}
/* Grid example */
.container {
display: grid;
grid-template-columns: 1fr 2fr;
}
Responsive web design is essential for providing an optimal user experience across a diverse range of devices, ensuring that your website looks and works great on all screens.
7.4 Best Practices and Coding Standards
Crafting High-Quality HTML Code
Adhering to best practices and coding standards is crucial for writing maintainable and accessible HTML code. Consistency and clean code not only make your work more manageable but also improve collaboration with other developers.
Indentation and Formatting
Consistent indentation and formatting make your code more readable and maintainable. Choose a style guide and stick to it.
<div>
<p>Indented content for clarity.</p>
</div>
Semantic HTML
Use semantic HTML elements to provide meaning and structure to your content. Semantic tags like <article>, <section>, and <nav> help both browsers and assistive technologies understand your page.
Accessibility
Ensure that your HTML is accessible by following accessibility guidelines. Use appropriate alt attributes for images, provide meaningful labels for form elements, and structure your content logically.
<img src="image.jpg" alt="A beautiful landscape">
Comments and Documentation
Add comments to your HTML code to explain complex sections or to provide context. Documentation helps other developers understand your code.
<!-- This section contains the main content of the page -->
<main>
<!-- The following div is used for layout purposes -->
<div class="container">
...
</div>
</main>
7.5 Browser Compatibility and Polyfills
Ensuring Cross-Browser Compatibility
Web developers face the challenge of making their websites work consistently across different web browsers, each with its quirks and capabilities. This requires testing and sometimes implementing polyfills to ensure functionality in older or less feature-rich browsers.
Browser Testing
Regularly test your website in various web browsers, including popular choices like Chrome, Firefox, Safari, and Microsoft Edge. Pay attention to older versions, as some users may still use them.
Polyfills
Polyfills are scripts that provide modern functionality in older browsers that lack support for certain features. They bridge the gap, enabling your website to function consistently across all browsers.
<script src="polyfill.js"></script>
Polyfills are particularly helpful when using modern HTML features like <details> and <summary> elements.
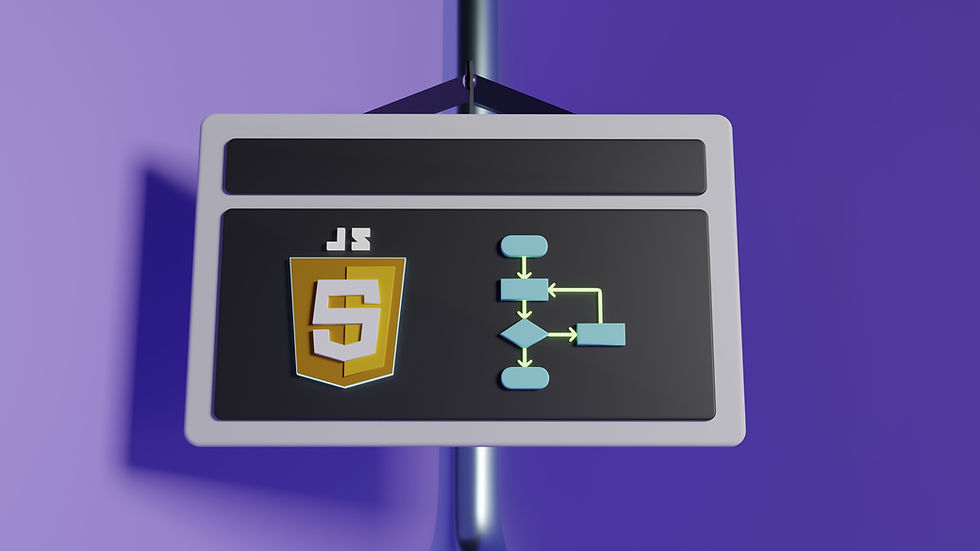
8. JavaScript and HTML Interaction
JavaScript is the dynamic force behind the interactivity and functionality of modern websites. In this exploration of JavaScript and its interaction with HTML, we dive deep into the fundamentals and techniques that empower you to create dynamic and engaging web experiences.
8.1 Introduction to JavaScript
The Language of the Web
JavaScript is a versatile and high-level programming language that is primarily used for adding interactivity and dynamic behaviour to websites. It is supported by all major web browsers, making it an integral part of web development.
Basic JavaScript Syntax
JavaScript code is typically embedded within HTML using <script> tags. Here's a simple example:
<script>
// JavaScript code goes here
console.log('Hello, World!');
</script>
In this example, the console.log statement outputs "Hello, World!" to the browser's console.
Variables and Data Types
JavaScript supports various data types, including numbers, strings, booleans, and objects. Variables are used to store and manipulate data.
let age = 30; // Number
let name = 'John'; // String
let isStudent = true; // Boolean
Functions
Functions are reusable blocks of code that perform specific tasks. They are defined using the function keyword.
function greet(name) {
return 'Hello, ' + name + '!';
}
Control Flow
JavaScript supports control flow structures like if, else, for, and while loops for making decisions and repeating actions.
f (age >= 18) {
console.log('You are an adult.');
} else {
console.log('You are a minor.');
}
JavaScript's rich syntax and powerful capabilities make it an essential language for building interactive web applications.
8.2 Embedding JavaScript in HTML
Integrating JavaScript with HTML
JavaScript can be included in an HTML document in several ways, depending on your requirements and preferences.
Inline JavaScript
You can embed JavaScript directly within an HTML document using the <script> element.
<script>
// JavaScript code goes here
console.log('Inline JavaScript');
</script>
Inline JavaScript is suitable for small scripts or when immediate execution is needed.
External JavaScript
For larger scripts and better code organization, you can include external JavaScript files using the <script> element's src attribute.
<script src="myscript.js"></script>
The myscript.js file contains your JavaScript code.
Asynchronous Loading
To improve page load times, you can use the async attribute to load external scripts asynchronously.
<script src="myscript.js" async></script>
This allows the rest of the page to load without waiting for the script to download and execute.
8.3 Event Handling
Interacting with User Actions
Event handling is a fundamental aspect of JavaScript, allowing you to respond to user interactions with web pages. Common events include clicks, keyboard inputs, mouse movements, and more.
Adding Event Listeners
You can attach event listeners to HTML elements to detect and respond to events.
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button clicked!');
});
In this example, when the button with the id "myButton" is clicked, an alert is displayed.
Event Object
Event handlers receive an event object that contains information about the event, such as the type of event, the target element, and more.
const button = document.getElementById('myButton');
button.addEventListener('click', function(event) {
console.log('Button clicked on', event.target);
});
Event Propagation
Events in the DOM propagate in two phases: capturing and bubbling. You can control the flow of events using the addEventListener method's third parameter (useCapture).
document.addEventListener('click', function() {
console.log('Document clicked');
},
true); // Use capture phase
Understanding event handling is crucial for creating interactive and responsive web applications.
8.4 Manipulating HTML with JavaScript
Dynamic Web Content
JavaScript allows you to manipulate HTML elements and their content dynamically. This capability is essential for creating interactive web applications.
Accessing Elements
You can access HTML elements using JavaScript by selecting them based on their attributes, such as id, class, or HTML tag.
const heading = document.getElementById('myHeading');
const paragraphs = document.getElementsByClassName('myParagraph');
const buttons = document.querySelectorAll('button');
Modifying Content
JavaScript enables you to change the content of HTML elements, including text, attributes, and even the structure of the DOM.
heading.textContent = 'New Heading';
paragraphs[0].innerHTML = 'Updated paragraph content';
Creating and Deleting Elements
JavaScript also allows you to create new HTML elements and remove existing ones.
const newDiv = document.createElement('div');
newDiv.textContent = 'New Div Element';
const parentElement = document.getElementById('parent');
parentElement.appendChild(newDiv);
const elementToRemove = document.getElementById('removeMe');
elementToRemove.remove();
Dynamic manipulation of HTML content with JavaScript is the foundation for creating engaging and interactive web applications.
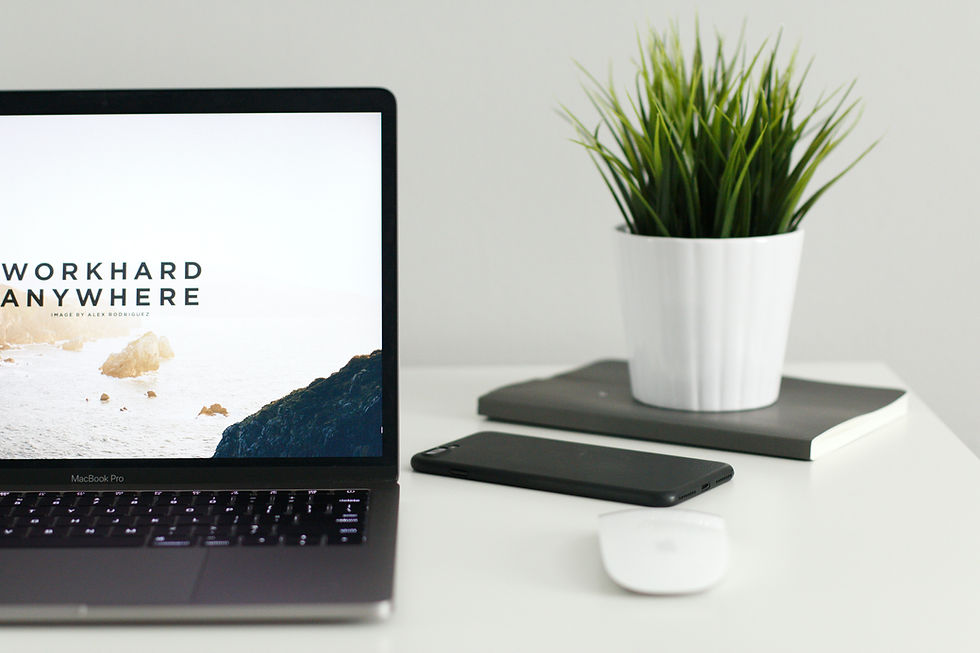
9. Building a Complete Web Page
Creating a complete web page involves combining HTML, CSS, and JavaScript to deliver a seamless and interactive user experience. In this guide, we'll embark on a project to create a personal website, covering everything from structuring the content with HTML to styling it with CSS and adding interactivity with JavaScript. Finally, we'll explore the process of deploying your web page for the world to see.
9.1 Project: Creating a Personal Website
Define Your Purpose
Before diving into code, clarify the purpose of your personal website. Are you building an online portfolio, a blog, or a personal landing page? Knowing your goals will guide your design and content decisions.
Planning Your Content
Identify the key sections and content you want to include on your website. Common sections for a personal website might include:
Home: An introduction and welcome message.
About: Information about yourself, including your background, skills, and interests.
Portfolio: Showcase your work, projects, or achievements.
Blog: Share your thoughts, articles, or updates.
Contact: Provide a way for visitors to get in touch with you.
9.2 Combining HTML, CSS, and JavaScript
Structuring Your HTML
Begin by structuring your website's content using HTML. Use semantic HTML elements to provide meaning to your content and improve accessibility.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Your Portfolio</title>
<!-- Include Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<!-- Include Custom CSS -->
<link rel="stylesheet" href="style.css">
</head>
<body>
<!-- Navbar -->
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<div class="container">
<a class="navbar-brand" href="#">Your Name</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav ml-auto">
<li class="nav-item">
<a class="nav-link" href="#about">About</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#portfolio">Portfolio</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#skills">Skills</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#experience">Experience</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#education">Education</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#blog">Blog</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#contact">Contact</a>
</li>
</ul>
</div>
</div>
</nav>
<!-- Hero Section -->
<section class="hero-section">
<div class="container">
<div class="row">
<div class="col-lg-6">
<h1 class="hero-title">Welcome to Your Portfolio</h1>
<p class="hero-description">Showcasing your amazing work and skills.</p>
</div>
</div>
</div>
</section>
<!-- About Section -->
<section id="about" class="about-section">
<div class="container">
<div class="row">
<div class="col-lg-6">
<h2 class="about-title">About Me</h2>
<p class="about-description">A brief description of yourself and your interests. Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
</div>
</div>
</div>
</section>
<!-- Portfolio Section -->
<section id="portfolio" class="portfolio-section">
<div class="container">
<h2 class="portfolio-title">Portfolio</h2>
<div class="row">
<!-- Portfolio Item 1 -->
<div class="col-lg-4 col-md-6 portfolio-item">
<div class="portfolio-card">
<img src="portfolio-item1.jpg" alt="Project 1" class="portfolio-image">
<div class="portfolio-details">
<h3 class="portfolio-item-title">Project 1</h3>
<p class="portfolio-item-description">Description of Project 1. Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
<a href="#" class="btn btn-primary portfolio-button">View Project</a>
</div>
</div>
</div>
<!-- Portfolio Item 2 -->
<div class="col-lg-4 col-md-6 portfolio-item">
<div class="portfolio-card">
<img src="portfolio-item2.jpg" alt="Project 2" class="portfolio-image">
<div class="portfolio-details">
<h3 class="portfolio-item-title">Project 2</h3>
<p class="portfolio-item-description">Description of Project 2. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<a href="#" class="btn btn-primary portfolio-button">View Project</a>
</div>
</div>
</div>
<!-- Portfolio Item 3 -->
<div class="col-lg-4 col-md-6 portfolio-item">
<div class="portfolio-card">
<img src="portfolio-item3.jpg" alt="Project 3" class="portfolio-image">
<div class="portfolio-details">
<h3 class="portfolio-item-title">Project 3</h3>
<p class="portfolio-item-description">Description of Project 3. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</p>
<a href="#" class="btn btn-primary portfolio-button">View Project</a>
</div>
</div>
</div>
</div>
</div>
</section>
<!-- Skills Section -->
<section id="skills" class="skills-section">
<div class="container">
<h2 class="skills-title">Skills</h2>
<div class="row">
<!-- Skill Category 1 -->
<div class="col-md-6">
<h3 class="skill-category">Web Development</h3>
<ul class="skill-list">
<li class="skill-list-item">HTML5</li>
<li class="skill-list-item">CSS3</li>
<li class="skill-list-item">JavaScript</li>
<li class="skill-list-item">React</li>
<!-- Add more skills as needed -->
</ul>
</div>
<!-- Skill Category 2 -->
<div class="col-md-6">
<h3 class="skill-category">Design Tools</h3>
<ul class="skill-list">
<li class="skill-list-item">Adobe Photoshop</li>
<li class="skill-list-item">Sketch</li>
</ul>
</div>
</div>
</div>
</section>
<!-- Experience Section -->
<section id="experience" class="experience-section">
<div class="container">
<h2 class="experience-title">Experience</h2>
<div class="row">
<!-- Experience Item 1 -->
<div class="col-md-6">
<div class="experience-item">
<h3 class="experience-title">Software Developer</h3>
<p class="experience-description">Company XYZ, January 2020 - Present</p>
<p class="experience-description">Description of your role and responsibilities.</p>
</div>
</div>
<!-- Experience Item 2 -->
<div class="col-md-6">
<div class="experience-item">
<h3 class="experience-title">Front-end Developer</h3>
<p class="experience-description">Company ABC, June 2018 - December 2019</p>
<p class="experience-description">Description of your role and achievements.</p>
</div>
</div>
</div>
</div>
</section>
<!-- Education Section -->
<section id="education" class="education-section bg-primary text-white">
<div class="container">
<h2 class="education-title">Education</h2>
<div class="row">
<!-- Education Item 1 -->
<div class="col-md-6">
<div class="education-item">
<h3 class="education-title">Bachelor's in Computer Science</h3>
<p class="education-description text-white">University of XYZ, Graduated in May 2017</p>
<p class="education-descriptiontext-white">Relevant coursework and achievements.</p>
</div>
</div>
<!-- Education Item 2 -->
<div class="col-md-6">
<div class="education-item">
<h3 class="education-title">Master's in Web Development</h3>
<p class="education-description text-white">Web Development Institute, Graduated in May 2019</p>
<p class="education-description text-white">Thesis or additional details about your studies.</p>
</div>
</div>
</div>
</div>
</section>
<!-- Blog Section -->
<section id="blog" class="blog-section">
<div class="container">
<h2 class="blog-title">Blog</h2>
<div class="row">
<!-- Blog Post 1 -->
<div class="col-lg-6">
<div class="blog-post">
<h3 class="blog-post-title">The Importance of Responsive Web Design</h3>
<p class="blog-post-date">Published on January 15, 2023</p>
<p class="blog-post-content">In this blog post, I explore the significance of responsive web design in the modern digital landscape. We'll dive into best practices and techniques for creating websites that adapt seamlessly to different screen sizes and devices. Responsive web design is essential for delivering an optimal user experience and improving your website's visibility on search engines.</p>
<a href="#" class="btn btn-primary blog-read-more">Read More</a>
</div>
</div>
<!-- Blog Post 2 -->
<div class="col-lg-6">
<div class="blog-post">
<h3 class="blog-post-title">Mastering CSS Grid Layouts</h3>
<p class="blog-post-date">Published on February 22, 2023</p>
<p class="blog-post-content">CSS Grid Layout is a powerful tool for creating complex and responsive web layouts. In this blog post, we'll delve into the world of CSS Grid and learn how to create advanced layouts with ease. You'll discover techniques for building flexible grids, aligning content, and achieving intricate designs. Whether you're a beginner or an experienced developer, mastering CSS Grid will elevate your web design skills.</p>
<a href="#" class="btn btn-primary blog-read-more">Read More</a>
</div>
</div>
</div>
</div>
</section>
<!-- Contact Section -->
<section id="contact" class="contact-section bg-light">
<div class="container">
<h2 class="contact-title">Contact Me</h2>
<div class="row">
<div class="col-lg-6">
<form id="contact-form" class="contact-form">
<div class="form-group">
<label for="name">Name</label>
<input type="text" id="name" name="name" class="form-control" required>
</div>
<div class="form-group">
<label for="email">Email</label>
<input type="email" id="email" name="email" class="form-control" required>
</div>
<div class="form-group">
<label for="message">Message</label>
<textarea id="message" name="message" class="form-control" rows="4" required></textarea>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
</div>
</div>
</section>
<!-- Footer Section -->
<footer class="footer-section">
<div class="container text-center">
<p>© 2023 Your Name. All rights reserved.</p>
</div>
</footer>
<!-- Include Bootstrap JS -->
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.5.3/dist/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
<!-- Include Custom JavaScript -->
<script src="script.js"></script>
</body>
</html>
Styling with CSS
Create a CSS file (styles.css) to define the visual style of your website. Use CSS to control layout, typography, colours, and other design aspects.
/* Reset some default styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
/* Global Styles */
body {
font-family: Arial, sans-serif;
background-color: #f8f8f8;
color: #333;
line-height: 1.6;
}
a {
text-decoration: none;
color: #007bff;
transition: color 0.3s ease;
}
a:hover {
color: #0056b3;
}
.container {
max-width: 1200px;
margin: 0 auto;
}
/* Navbar Styles */
.navbar {
background-color: #333;
padding: 1rem 0;
}
.navbar-brand {
font-size: 1.5rem;
font-weight: bold;
}
.navbar-toggler-icon {
background-color: #fff;
}
.navbar-nav .nav-link {
color: #fff;
font-size: 1rem;
padding: 0.5rem 1rem;
transition: background-color 0.3s ease;
}
.navbar-nav .nav-link:hover {
background-color: #007bff;
border-radius: 0.25rem;
}
/* Hero Section Styles */
.hero-section {
background-image: linear-gradient(rgba(0, 0, 0, 0.5), rgba(0, 0, 0, 0.5)), url('hero-bg.jpg');
background-size: cover;
background-position: center;
text-align: center;
color: #fff;
padding: 4rem 0;
}
.hero-title {
font-size: 3rem;
margin-bottom: 1rem;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.5);
animation: fadeInUp 1s ease;
}
@keyframes fadeInUp {
from {
opacity: 0;
transform: translateY(20px);
}
to {
opacity: 1;
transform: translateY(0);
}
}
.hero-description {
font-size: 1.2rem;
margin-bottom: 2rem;
animation: fadeInUp 1s ease 0.5s;
}
/* About Section Styles */
.about-section {
padding: 4rem 0;
}
.about-title {
font-size: 2.5rem;
margin-bottom: 1rem;
}
.about-description {
font-size: 1.1rem;
color: #555;
animation: fadeInLeft 1s ease;
}
@keyframes fadeInLeft {
from {
opacity: 0;
transform: translateX(-20px);
}
to {
opacity: 1;
transform: translateX(0);
}
}
/* Portfolio Section Styles */
.portfolio-section {
padding: 4rem 0;
}
.portfolio-title {
font-size: 2.5rem;
margin-bottom: 2rem;
}
.portfolio-item {
margin-bottom: 2rem;
transition: transform 0.3s ease;
}
.portfolio-item:hover {
transform: scale(1.03);
}
.portfolio-card {
background-color: #fff;
border: 1px solid #eee;
padding: 1.5rem;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
transition: box-shadow 0.3s ease;
}
.portfolio-image {
width: 100%;
height: auto;
border-radius: 0.25rem;
}
.portfolio-item-title {
font-size: 1.5rem;
margin: 1rem 0;
}
.portfolio-item-description {
font-size: 1rem;
color: #555;
margin-bottom: 1rem;
}
.portfolio-button {
display: inline-block;
margin-top: 1rem;
background-color: #007bff;
color: #fff;
padding: 0.5rem 1rem;
border-radius: 0.25rem;
text-align: center;
text-decoration: none;
transition: background-color 0.3s ease;
}
.portfolio-button:hover {
background-color: #0056b3;
}
/* Skills Section Styles */
.skills-section {
padding: 4rem 0;
}
.skills-title {
font-size: 2.5rem;
margin-bottom: 2rem;
}
.skill-category {
font-size: 1.5rem;
margin-bottom: 1rem;
}
.skill-list {
list-style-type: none;
padding: 0;
}
.skill-list-item {
font-size: 1.1rem;
margin-bottom: 0.5rem;
position: relative;
padding-left: 20px;
}
.skill-list-item::before {
content: '\2022';
position: absolute;
left: 0;
color: #007bff;
}
/* Experience Section Styles */
.experience-section {
padding: 4rem 0;
}
.experience-title {
font-size: 2.5rem;
margin-bottom: 2rem;
}
.experience-item {
margin-bottom: 2rem;
}
.experience-title {
font-size: 1.5rem;
margin-bottom: 1rem;
}
.experience-description {
font-size: 1.1rem;
color: #555;
}
/* Education Section Styles */
.education-section {
padding: 4rem 0;
}
.education-title {
font-size: 2.5rem;
margin-bottom: 2rem;
}
.education-item {
margin-bottom: 2rem;
}
.education-title {
font-size: 1.5rem;
margin-bottom: 1rem;
}
.education-description {
font-size: 1.1rem;
color: #555;
}
/* Blog Section Styles */
.blog-section {
padding: 4rem 0;
}
.blog-title {
font-size: 2.5rem;
margin-bottom: 2rem;
}
.blog-post {
background-color: #fff;
border: 1px solid #eee;
padding: 2rem;
margin-bottom: 2rem;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
transition: transform 0.3s ease;
}
.blog-post:hover {
transform: translateY(-5px);
}
.blog-post-title {
font-size: 1.5rem;
margin-bottom: 1rem;
}
.blog-post-date {
font-size: 1rem;
color: #777;
margin-bottom: 1rem;
}
.blog-post-content {
font-size: 1.1rem;
color: #555;
margin-bottom: 1rem;
}
.blog-read-more {
display: inline-block;
margin-top: 1rem;
background-color: #007bff;
color: #fff;
padding: 0.5rem 1rem;
border-radius: 0.25rem;
text-align: center;
text-decoration: none;
transition: background-color 0.3s ease;
}
.blog-read-more:hover {
background-color: #0056b3;
}
/* Contact Section Styles */
.contact-section {
padding: 4rem 0;
}
.contact-title {
font-size: 2.5rem;
margin-bottom: 2rem;
}
.contact-form {
background-color: #fff;
border: 1px solid #eee;
padding: 2rem;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.form-group {
margin-bottom: 1.5rem;
}
.form-label {
font-size: 1.2rem;
}
.form-control {
width: 100%;
padding: 0.5rem;
font-size: 1rem;
border: 1px solid #ccc;
border-radius: 0.25rem;
transition: border-color 0.3s ease, box-shadow 0.3s ease;
}
.form-control:focus {
border-color: #007bff;
box-shadow: 0 0 5px rgba(0, 123, 255, 0.5);
}
/* Footer Section Styles */
.footer-section {
background-color: #333;
color: #fff;
padding: 2rem 0;
text-align: center;
}
/* Media Queries for Responsiveness */
@media (max-width: 768px) {
.hero-title {
font-size: 2rem;
}
.hero-description {
font-size: 1rem;
}
}
Adding Interactivity with JavaScript
JavaScript enhances the user experience by adding interactivity and dynamic behaviour. Use JavaScript to respond to user actions, validate forms, or load content dynamically.
document.addEventListener('DOMContentLoaded', function () {
const contactForm = document.querySelector('.contact-form');
const submitButton = contactForm.querySelector('button[type="submit"]');
contactForm.addEventListener('submit', function (e) {
e.preventDefault();
// Validate the form fields
const nameInput = contactForm.querySelector('input[name="name"]');
const emailInput = contactForm.querySelector('input[name="email"]');
const messageInput = contactForm.querySelector('textarea[name="message"]');
const nameValue = nameInput.value.trim();
const emailValue = emailInput.value.trim();
const messageValue = messageInput.value.trim();
// Regular expression for a valid email format
const emailPattern = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/;
const errors = {
name: [],
email: [],
message: []
};
// Validate name field
if (nameValue === '') {
errors.name.push('Please enter your name.');
}
// Validate email field
if (emailValue === '') {
errors.email.push('Please enter your email.');
} else if (!emailPattern.test(emailValue)) {
errors.email.push('Please enter a valid email address.');
}
// Validate message field
if (messageValue === '') {
errors.message.push('Please enter your message.');
}
// Display error messages if any
displayErrors(errors);
// If there are no errors, submit the form
if (Object.keys(errors).every(field => errors[field].length === 0)) {
alert('Form submitted successfully!');
contactForm.reset();
}
});
// Function to display error messages
function displayErrors(errors) {
for (const field in errors) {
const errorContainer = document.querySelector(`.${field}-error`);
errorContainer.innerHTML = '';
if (errors[field].length > 0) {
errors[field].forEach(message => {
const errorItem = document.createElement('li');
errorItem.textContent = message;
errorContainer.appendChild(errorItem);
});
}
}
}
});
In this example, JavaScript adds smooth scrolling to anchor links in the navigation menu.
9.3 Deploying Your Web Page
Hosting and Domain
To make your website accessible on the internet, you'll need a hosting service and a domain name. Popular hosting providers include GitHub Pages, Netlify, and Vercel. Register a domain name that reflects your website's identity and purpose.
Uploading Your Files
Once you have hosting and a domain, upload your HTML, CSS, JavaScript, and other assets to your hosting provider's server. Most hosting services offer user-friendly interfaces or command-line tools for this purpose.
Testing and Optimization
Before launching, thoroughly test your website across various browsers and
devices to ensure compatibility. Optimize images and assets for faster loading times.
Launch and Promote
With everything in place, it's time to launch your website. Share it with friends, colleagues, and on social media to promote your online presence. Consider implementing SEO techniques to improve your website's discoverability in search engines.
Ongoing Maintenance
A website is a living entity. Regularly update your content, fix any issues that arise, and keep your website secure with software updates and security best practices.
10. Conclusion and Further Learning
As we reach the conclusion of this comprehensive guide on HTML essentials and your journey through web development, let's take a moment to recap what you've learned, discuss how to continue your growth as a web developer, and explore valuable resources for further reading and learning.
10.1 Recap of HTML Essentials
The Foundation of Web Development
HTML, Hypertext Markup Language, is the fundamental language that powers the World Wide Web. Throughout this guide, you've delved into the core concepts of HTML, learning how to structure web content, create links, include images, and build tables. Here's a brief recap of the essential HTML elements and concepts you've covered:
Document Structure
HTML documents are structured with opening and closing tags, forming a tree-like hierarchy.
The <html> element is the root of every HTML document.
The <head> section contains metadata, including the page title, character encoding, and links to external resources.
The <body> section contains the visible content of the web page.
Text and Headings
Headings are defined using <h1> to <h6> elements, representing different levels of importance.
Paragraphs are created with the <p> element.
Text can be emphasized with <em> (emphasized) and <strong> (strongly emphasized) tags.
Lists
Unordered lists are created with <ul> and list items with <li>.
Ordered lists are created with <ol> and list items with <li>.
Links
Hyperlinks are created using the <a> element.
The href attribute specifies the target URL.
Anchor links allow navigation within the same page using the # symbol.
Images
Images are displayed using the <img> element, with the src attribute pointing to the image file.
The alt attribute provides alternative text for accessibility and SEO.
Tables
Tables are constructed with the <table> element.
Rows are defined with <tr> (table row), and data cells with <td> (table data).
Table headers are created using <th> (table header) elements.
Forms
Forms are created with the <form> element.
Input fields can be text, checkboxes, radio buttons, and more.
The label element associates a label with an input field for better accessibility.
Semantic HTML
Semantic HTML elements like <header>, <nav>, <main>, and <footer> provide meaning and structure to web content.
They improve accessibility and search engine optimization (SEO).
10.2 Continuing Your Web Development Journey
Building on the Foundations
While you've gained a strong understanding of HTML, web development is a vast field that continues to evolve. To further your journey and become a proficient web developer, consider the following steps:
1. Learn CSS
HTML provides the structure, but Cascading Style Sheets (CSS) are the key to designing visually appealing and responsive websites. Dive into CSS to control layout, typography, colors, and animations.
2. Master JavaScript
JavaScript is the language of interactivity on the web. Learn JavaScript to add dynamic behavior, create interactive forms, and build web applications.
3. Explore Front-End Frameworks
Front-end frameworks like React, Angular, and Vue.js streamline the development of complex web applications. Choose one that aligns with your goals and delve deeper into it.
4. Understand Back-End Development
For full-stack development, learn a server-side language like Node.js, Python, or Ruby. This will enable you to create server-side logic, handle databases, and build complete web applications.
5. Study Responsive Design
With the increasing variety of devices and screen sizes, mastering responsive design is essential. Learn how to create websites that adapt gracefully to different devices.
6. Explore Web Accessibility
Web accessibility ensures that your websites are usable by people with disabilities. Delve into accessibility guidelines and practices to make your websites inclusive.
7. Version Control
Learn how to use version control systems like Git. It's crucial for collaborating with other developers and managing code changes.
8. Project-Based Learning
Practice what you've learned by taking on real-world projects. Build personal websites, web apps, or contribute to open-source projects.
10.3 Resources and Further Reading
Your Learning Journey Continues
To support your ongoing learning in web development, here are some valuable resources and further reading materials:
Books
Online Courses and Tutorials
Codecademy (codecademy.com)
freeCodeCamp (freecodecamp.org)
MDN Web Docs (developer.mozilla.org)
Coursera (coursera.org)
Udemy (udemy.com)
Coding Practice
LeetCode (leetcode.com)
HackerRank (hackerrank.com)
Project Euler (projecteuler.net)
Web Development Communities
Stack Overflow (stackoverflow.com)
GitHub (github.com)
Reddit r/webdev (reddit.com/r/webdev)
Forums and Blogs
Smashing Magazine (smashingmagazine.com)
A List Apart (alistapart.com)
CSS-Tricks (css-tricks.com)
SitePoint (sitepoint.com)
Web Development Tools
Visual Studio Code (code.visualstudio.com)
Chrome DevTools (developers.google.com/web/tools/chrome-devtools)
Web Standards and Documentation
W3C (w3.org)
WHATWG (whatwg.org)
WebAIM (webaim.org)
Remember that web development is a continuously evolving field. Stay curious, keep exploring, and embrace lifelong learning as you embark on your journey to becoming a proficient web developer. With dedication and a passion for building the web, you'll contribute to the digital world's ever-growing landscape. Happy coding!
Informative and helpful for a beginner!